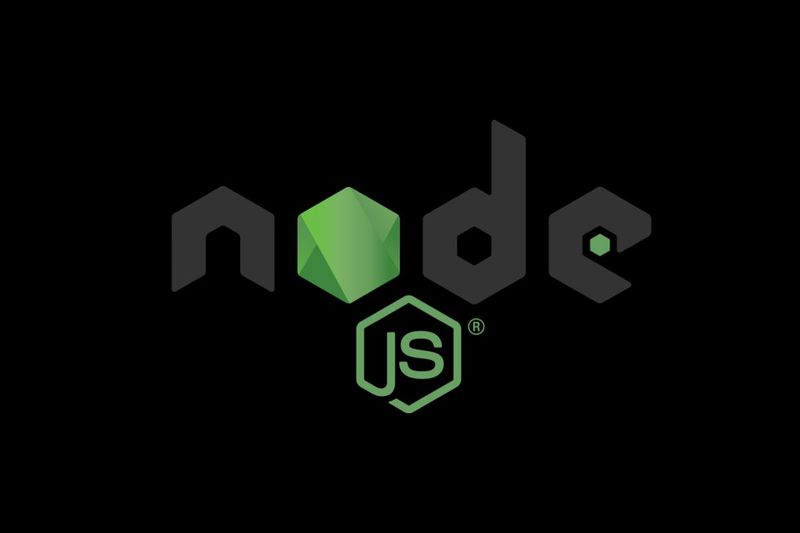
Node.js is a powerful JavaScript runtime that enables developers to build scalable and efficient applications. One of the key features of Node.js is its modular architecture, which allows developers to organize code into reusable units known as modules. Additionally, NPM (Node Package Manager) provides a robust ecosystem for managing dependencies.
In this guide, we’ll explore:
- Built-in modules (fs, path, os, etc.)
- Creating and using custom modules
- Import/export (CommonJS vs ES Modules)
- NPM (Node Package Manager)
- Managing dependencies in package.json
1. Built-in Modules in Node.js
Node.js comes with a set of core modules that provide essential functionalities without requiring external libraries. Some commonly used built-in modules include:
a) File System (fs) Module
The fs
module allows interaction with the file system.
Example: Reading a File
const fs = require('fs'); fs.readFile('example.txt', 'utf8', (err, data) => { if (err) throw err; console.log(data); });
b) Path Module
The path
module helps in handling and transforming file paths.
Example: Joining Paths
const path = require('path'); const filePath = path.join(__dirname, 'folder', 'file.txt'); console.log(filePath);
c) OS Module
The os
module provides operating system-related utilities.
Example: Getting System Information
const os = require('os'); console.log(`OS Platform: ${os.platform()}`); console.log(`Total Memory: ${os.totalmem()} bytes`);
Other useful built-in modules include http
(for creating servers), crypto
(for cryptography), and events
(for event handling).
2. Creating and Using Custom Modules
Node.js allows developers to create custom modules to structure their applications better. A module in Node.js is simply a JavaScript file that exports functions, objects, or variables.
a) Creating a Custom Module
Create a file called math.js
:
function add(a, b) { return a + b; } module.exports = { add };
b) Importing and Using a Custom Module
In another file (app.js
), import the custom module:
const math = require('./math'); console.log(math.add(5, 3)); // Output: 8
3. Import/Export: CommonJS vs ES Modules
Node.js supports two module systems:
- CommonJS (CJS) – Uses
require()
andmodule.exports
. - ES Modules (ESM) – Uses
import
andexport
statements.
a) CommonJS (Default in Node.js)
// math.js module.exports = { add: (a, b) => a + b }; // app.js const math = require('./math'); console.log(math.add(2, 3));
b) ES Modules (ESM)
To enable ES modules, add "type": "module"
in package.json
.
// math.js export function add(a, b) { return a + b; } // app.js import { add } from './math.js'; console.log(add(2, 3));
Use CommonJS for backward compatibility and ESM for modern JavaScript development.
4. NPM (Node Package Manager)
NPM is the default package manager for Node.js and provides access to thousands of libraries.
a) Initializing a Project
To start using NPM, initialize a project:
npm init -y
This generates a package.json
file, which stores project metadata and dependencies.
b) Installing Dependencies
To install a package, use:
npm install lodash
This installs lodash
and adds it to package.json
.
c) Installing as a Dev Dependency
For packages required only during development:
npm install nodemon --save-dev
d) Global Installation
Some packages are installed globally for CLI usage:
npm install -g typescript
5. Managing Dependencies in package.json
The package.json
file contains:
{ "name": "my-project", "version": "1.0.0", "dependencies": { "express": "^4.18.2" }, "devDependencies": { "nodemon": "^2.0.20" } }
a) Removing Dependencies
To remove a package:
npm uninstall lodash
b) Updating Dependencies
To update all packages:
npm update
Conclusion
Understanding Node.js modules and NPM is essential for efficient development. Built-in modules provide fundamental capabilities, while custom modules allow better project structure. Knowing the difference between CommonJS and ES Modules helps in writing maintainable code, and using NPM efficiently ensures seamless package management.
By mastering these concepts, you’ll be well-equipped to build and manage scalable Node.js applications.
Leave a Comment